In this tutorial, we show how to pull products in JSON format through API in Opencart. First, read the post below to understand the OpenCart API:
We take an example between two servers one from https://webocreation.com/nepalbuddha which acts as Server Responder of API which is built in OpenCart and https://tuliprsdynasty.com/api/ as the requestor.
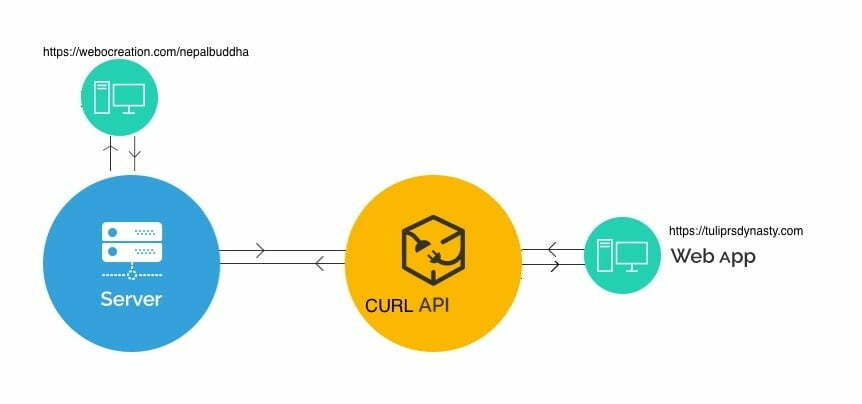
Responding server
OpenCart does not provide API to show the products by default so we need to make some changes in responding server to show all products. For that in your responding server go to catalog/controller/api/ and create
load->language('api/cart'); $this->load->model('catalog/product'); $this->load->model('tool/image'); $json = array(); $json['products'] = array(); $filter_data = array(); $results = $this->model_catalog_product->getProducts($filter_data); foreach ($results as $result) { if ($result['image']) { $image = $this->model_tool_image->resize($result['image'], $this->config->get('theme_' . $this->config->get('config_theme') . '_image_product_width'), $this->config->get('theme_' . $this->config->get('config_theme') . '_image_product_height')); } else { $image = $this->model_tool_image->resize('placeholder.png', $this->config->get('theme_' . $this->config->get('config_theme') . '_image_product_width'), $this->config->get('theme_' . $this->config->get('config_theme') . '_image_product_height')); } if ($this->customer->isLogged() || !$this->config->get('config_customer_price')) { $price = $this->currency->format($this->tax->calculate($result['price'], $result['tax_class_id'], $this->config->get('config_tax')), $this->session->data['currency']); } else { $price = false; } if ((float) $result['special']) { $special = $this->currency->format($this->tax->calculate($result['special'], $result['tax_class_id'], $this->config->get('config_tax')), $this->session->data['currency']); } else { $special = false; } if ($this->config->get('config_tax')) { $tax = $this->currency->format((float) $result['special'] ? $result['special'] : $result['price'], $this->session->data['currency']); } else { $tax = false; } if ($this->config->get('config_review_status')) { $rating = (int) $result['rating']; } else { $rating = false; } $data['products'][] = array( 'product_id' => $result['product_id'], 'thumb' => $image, 'name' => $result['name'], 'description' => utf8_substr(trim(strip_tags(html_entity_decode($result['description'], ENT_QUOTES, 'UTF-8'))), 0, $this->config->get('theme_' . $this->config->get('config_theme') . '_product_description_length')) . '..', 'price' => $price, 'special' => $special, 'tax' => $tax, 'minimum' => $result['minimum'] > 0 ? $result['minimum'] : 1, 'rating' => $result['rating'], 'href' => $this->url->link('product/product', 'product_id=' . $result['product_id']), ); } $json['products'] = $data['products']; $this->response->addHeader('Content-Type: application/json'); $this->response->setOutput(json_encode($json)); } }
With the above code it will provide you directly accessing API URL index.php?route=api/product. As per our example, it is https://webocreation.com/nepalbuddha/index.php?route=api/product. Here we did not do any authentication and authorization. It directly shows all products whoever is called from that URL. In our upcoming post, we will show how to show categories to API users only.
Requesting server
In your requesting server, create a file lets say apiproducts.php, as per our example let's say we create https://tuliprsdynasty.com/api/apiproducts.php In this file let's make a CURL request to the get the products: https://webocreation.com/nepalbuddha/index.php?route=api/product. Following is the code:
$url . "/index.php?route=api%2Fproduct",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => array(
"cache-control: no-cache",
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
echo $response;
}
Finally, the response you get will be like below:
{
"products": [{
"product_id": "30",
"thumb": "https:\/\/webocreation.com\/nepalbuddha\/image\/cache\/catalog\/demo\/canon_eos_5d_1-228x228.jpg",
"name": "Canon EOS 5D",
"description": "Canon's press material for the EOS 5D states that it 'defines (a) new D-SLR category', while we're n..",
"price": "$122.00",
"special": "$98.00",
"tax": "$80.00",
"minimum": "1",
"rating": 0,
"href": "https:\/\/webocreation.com\/nepalbuddha\/index.php?route=product\/product&product_id=30"
}, {
"product_id": "47",
"thumb": "https:\/\/webocreation.com\/nepalbuddha\/image\/cache\/catalog\/demo\/hp_1-228x228.jpg",
"name": "HP LP3065",
"description": "Stop your co-workers in their tracks with the stunning new 30-inch diagonal HP LP3065 Flat Panel Mon..",
"price": "$122.00",
"special": false,
"tax": "$100.00",
"minimum": "1",
"rating": 0,
"href": "https:\/\/webocreation.com\/nepalbuddha\/index.php?route=product\/product&product_id=47"
}]
}
As per our API set up, it just returns product_id, thumb, name, description, price, special, tax, minimum, rating, and link of the product. If you want to make changes then you have to make changes in catalog/controller/api/product.php as per your need. In this
You can check following Opencart API related posts:
- https://webocreation.com/opencart-api-documentation-to-create-read-query-update-and-upsert
- https://webocreation.com/opencart-api-documentation-developer
Please don’t forget to post your questions or comments so that we can add extra topics. Likewise, you can follow us at our twitter account @rupaknpl and subscribe to our YouTube channel for Opencart tutorials.